Write a Program. That Reads a Word and Print the Word in Reverse
Are you lot diving deeper into Python lists and wanting to learn about dissimilar ways to reverse them? If so, then this tutorial is for you. Here, y'all'll acquire near a few Python tools and techniques that are handy when it comes to reversing lists or manipulating them in reverse gild. This knowledge will complement and improve your listing-related skills and brand you more proficient with them.
In this tutorial, you'll learn how to:
- Reverse existing lists in identify using
.reverse()
and other techniques - Create reversed copies of existing lists using
reversed()
and slicing - Use iteration, comprehensions, and recursion to create reversed lists
- Iterate over your lists in reverse order
- Sort your lists in contrary order using
.sort()
andsorted()
To get the most out of this tutorial, it would be helpful to know the basics of iterables, for
loops, lists, listing comprehensions, generator expressions, and recursion.
Reversing Python Lists
Sometimes y'all demand to procedure Python lists starting from the last element down to the first—in other words, in reverse social club. In full general, there are two main challenges related to working with lists in reverse:
- Reversing a list in place
- Creating reversed copies of an existing list
To encounter the first claiming, you lot can utilize either .opposite()
or a loop that swaps items by index. For the 2nd, yous tin can apply reversed()
or a slicing operation. In the adjacent sections, you'll learn near different ways to attain both in your code.
Reversing Lists in Place
Like other mutable sequence types, Python lists implement .reverse()
. This method reverses the underlying listing in place for retention efficiency when you're reversing big list objects. Here's how you can apply .reverse()
:
>>>
>>> digits = [ 0 , 1 , 2 , 3 , 4 , 5 , vi , seven , eight , ix ] >>> digits . reverse () >>> digits [9, 8, 7, 6, 5, 4, 3, 2, 1, 0]
When you call .contrary()
on an existing list, the method reverses it in identify. This fashion, when you admission the listing again, y'all get it in opposite gild. Note that .reverse()
doesn't return a new list but None
:
>>>
>>> digits = [ 0 , i , ii , 3 , 4 , 5 , 6 , 7 , 8 , 9 ] >>> reversed_digits = digits . reverse () >>> reversed_digits is None Truthful
Trying to assign the return value of .reverse()
to a variable is a common mistake related to using this method. The intent of returning None
is to remind its users that .reverse()
operates by side effect, changing the underlying list.
Okay! That was quick and straightforward! Now, how can yous contrary a list in place by hand? A mutual technique is to loop through the first half of information technology while swapping each element with its mirror analogue on the 2d half of the listing.
Python provides zero-based positive indices to walk sequences from left to right. It also allows you to navigate sequences from right to left using negative indices:
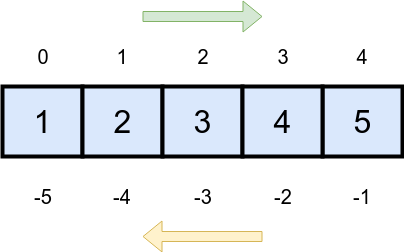
This diagram shows that you can access the get-go element of the list (or sequence) using either 0
or -5
with the indexing operator, like in sequence[0]
and sequence[-5]
, respectively. Yous can use this Python feature to reverse the underlying sequence in place.
For example, to opposite the list represented in the diagram, you tin can loop over the first half of the list and bandy the element at index 0
with its mirror at index -1
in the beginning iteration. Then y'all can switch the chemical element at index ane
with its mirror at index -2
and and so on until you get the list reversed.
Here'southward a representation of the whole process:
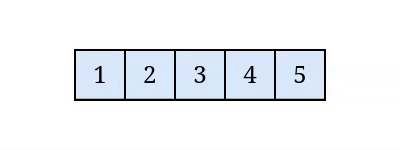
To translate this process into code, you tin can apply a for
loop with a range
object over the get-go half of the list, which you can get with len(digits) // two
. And so you can use a parallel consignment argument to swap the elements, like this:
>>>
>>> digits = [ 0 , i , 2 , 3 , 4 , five , six , seven , 8 , 9 ] >>> for i in range ( len ( digits ) // 2 ): ... digits [ i ], digits [ - 1 - i ] = digits [ - 1 - i ], digits [ i ] ... >>> digits [9, viii, 7, 6, v, 4, 3, ii, 1, 0]
This loop iterates through a range
object that goes from 0
to len(digits) // 2
. Each iteration swaps an item from the first half of the list with its mirror counterpart in the 2d half. The expression -1 - i
inside the indexing operator, []
, guarantees admission to the mirror particular. You lot can also employ the expression -i * (i + ane)
to provide the corresponding mirror index.
Besides the above algorithm, which takes advantage of index substitution, there are a few different ways to reverse lists by mitt. For example, you tin can utilize .pop()
and .insert()
like this:
>>>
>>> digits = [ 0 , 1 , 2 , 3 , 4 , five , 6 , 7 , eight , 9 ] >>> for i in range ( len ( digits )): ... last_item = digits . popular () ... digits . insert ( i , last_item ) ... >>> digits [9, 8, 7, half dozen, 5, iv, 3, 2, 1, 0]
In the loop, you telephone call .pop()
on the original listing without arguments. This call removes and returns the last item in the list, then you tin can store it in last_item
. So .insert()
moves last_item
to the position at index i
.
For example, the first iteration removes 9
from the right cease of the list and stores it in last_item
. Then it inserts 9
at index 0
. The side by side iteration takes eight
and moves it to alphabetize 1
, and so on. At the end of the loop, you become the list reversed in place.
Creating Reversed Lists
If you lot want to create a reversed re-create of an existing list in Python, and so y'all can employ reversed()
. With a list every bit an argument, reversed()
returns an iterator that yields items in contrary order:
>>>
>>> digits = [ 0 , 1 , ii , 3 , iv , 5 , half dozen , 7 , 8 , ix ] >>> reversed_digits = reversed ( digits ) >>> reversed_digits <list_reverseiterator object at 0x7fca9999e790> >>> list ( reversed_digits ) [9, eight, 7, 6, 5, 4, iii, 2, 1, 0]
In this instance, you call reversed()
with digits
as an argument. Then you store the resulting iterator in reversed_digits
. The call to list()
consumes the iterator and returns a new list containing the aforementioned items every bit digits
but in reverse society.
An important bespeak to note when you're using reversed()
is that it doesn't create a copy of the input list, and so changes on information technology affect the resulting iterator:
>>>
>>> fruits = [ "apple tree" , "banana" , "orange" ] >>> reversed_fruit = reversed ( fruits ) # Go the iterator >>> fruits [ - 1 ] = "kiwi" # Modify the concluding particular >>> next ( reversed_fruit ) # The iterator sees the change 'kiwi'
In this example, you call reversed()
to go the corresponding iterator over the items in fruits
. So you modify the last fruit. This change affects the iterator. You can confirm that by calling next()
to get the first item in reversed_fruit
.
If you demand to go a copy of fruits
using reversed()
, then you can call list()
:
>>>
>>> fruits = [ "apple" , "banana" , "orangish" ] >>> listing ( reversed ( fruits )) ['orange', 'assistant', 'apple']
As yous already know, the telephone call to list()
consumes the iterator that results from calling reversed()
. This mode, yous create a new listing equally a reversed re-create of the original one.
Python 2.iv added reversed()
, a universal tool to facilitate reverse iteration over sequences, as stated in PEP 322. In general, reversed()
can accept any objects that implement a .__reversed__()
method or that back up the sequence protocol, consisting of the .__len__()
and .__getitem__()
special methods. So, reversed()
isn't limited to lists:
>>>
>>> list ( reversed ( range ( ten ))) [ix, 8, 7, 6, 5, four, three, 2, 1, 0] >>> list ( reversed ( "Python" )) ['n', 'o', 'h', 't', 'y', 'P']
Here, instead of a list, you pass a range
object and a string as arguments to reversed()
. The function does its chore as expected, and you lot get a reversed version of the input data.
Another important point to highlight is that you tin can't utilise reversed()
with capricious iterators:
>>>
>>> digits = iter ([ 0 , i , 2 , 3 , 4 , 5 , half dozen , 7 , eight , 9 ]) >>> reversed ( digits ) Traceback (most recent phone call last): File "<stdin>", line ane, in <module> TypeError: 'list_iterator' object is non reversible
In this example, iter()
builds an iterator over your list of numbers. When y'all call reversed()
on digits
, you get a TypeError
.
Iterators implement the .__next__()
special method to walk through the underlying data. They're also expected to implement the .__iter__()
special method to render the current iterator case. However, they're not expected to implement either .__reversed__()
or the sequence protocol. And then, reversed()
doesn't work for them. If yous ever need to reverse an iterator similar this, then you should first convert it to a list using list()
.
Some other betoken to note is that you can't employ reversed()
with unordered iterables:
>>>
>>> digits = { 0 , one , ii , three , 4 , 5 , six , 7 , 8 , nine } >>> reversed ( digits ) Traceback (most recent telephone call last): File "<stdin>", line one, in <module> TypeError: 'set' object is not reversible
In this example, when yous attempt to apply reversed()
with a set
object, you get a TypeError
. This is considering sets don't proceed their items ordered, and so Python doesn't know how to contrary them.
Reversing Lists Through Slicing
Since Python 1.4, the slicing syntax has had a third argument, chosen stride
. However, that syntax initially didn't work on built-in types, such as lists, tuples, and strings. Python 2.3 extended the syntax to congenital-in types, and then you tin can use step
with them now. Here'southward the total-diddled slicing syntax:
This syntax allows y'all to extract all the items in a_list
from start
to stop − 1
by step
. The third offset, stride
, defaults to 1
, which is why a normal slicing functioning extracts the items from left to right:
>>>
>>> digits = [ 0 , 1 , 2 , iii , 4 , 5 , 6 , seven , viii , ix ] >>> digits [ i : 5 ] [ane, two, 3, 4]
With [i:v]
, you lot get the items from index i
to alphabetize 5 - one
. The particular with the index equal to stop
is never included in the final result. This slicing returns all the items in the target range considering step
defaults to ane
.
If you utilize a different step
, and then the slicing jumps equally many items as the value of pace
:
>>>
>>> digits = [ 0 , 1 , two , 3 , 4 , 5 , 6 , 7 , 8 , 9 ] >>> digits [ 0 :: two ] [0, 2, 4, 6, 8] >>> digits [:: 3 ] [0, 3, 6, ix]
In the outset example, [0::2]
extracts all items from index 0
to the cease of digits
, jumping over ii items each time. In the 2nd example, the slicing jumps 3
items every bit it goes. If you don't provide values to showtime
and stop
, and so they are set to 0
and to the length of the target sequence, respectively.
If you ready step
to -1
, so you get a slice with the items in contrary order:
>>>
>>> digits = [ 0 , 1 , 2 , three , 4 , 5 , 6 , seven , 8 , 9 ] >>> # Set step to -1 >>> digits [ len ( digits ) - 1 :: - ane ] [9, 8, seven, 6, v, 4, 3, 2, i, 0] >>> digits [0, one, 2, 3, 4, 5, half dozen, 7, 8, 9]
This slicing returns all the items from the correct stop of the list (len(digits) - i
) dorsum to the left end because you omit the second get-go. The residue of the magic in this instance comes from using a value of -ane
for pace
. When yous run this trick, you get a copy of the original list in opposite order without affecting the input data.
If you fully rely on implicit offsets, then the slicing syntax gets shorter, cleaner, and less error-prone:
>>>
>>> digits = [ 0 , 1 , ii , iii , 4 , five , half-dozen , seven , 8 , 9 ] >>> # Rely on default kickoff values >>> digits [:: - 1 ] [9, eight, 7, 6, 5, four, 3, 2, 1, 0]
Here, you lot ask Python to requite you the complete list ([::-1]
) but going over all the items from back to front past setting step
to -ane
. This is pretty neat, but reversed()
is more efficient in terms of execution time and retentiveness usage. It'south also more readable and explicit. So these are points to consider in your code.
Another technique to create a reversed copy of an existing listing is to use slice()
. The signature of this congenital-in function is like this:
This function works similarly to the indexing operator. It takes 3 arguments with like meaning to those used in the slicing operator and returns a slice object representing the gear up of indices returned by range(start, cease, pace)
. That sounds complicated, then hither are some examples of how slice()
works:
>>>
>>> digits = [ 0 , ane , 2 , three , iv , 5 , 6 , 7 , 8 , 9 ] >>> slice ( 0 , len ( digits )) slice(0, ten, None) >>> digits [ piece ( 0 , len ( digits ))] [0, ane, ii, 3, iv, 5, 6, 7, viii, 9] >>> slice ( len ( digits ) - 1 , None , - 1 ) slice(9, None, -1) >>> digits [ piece ( len ( digits ) - i , None , - 1 )] [9, 8, 7, 6, 5, 4, iii, 2, 1, 0]
The first call to slice()
is equivalent to [0:len(digits)]
. The 2d call works the same as [len(digits) - 1::-1]
. You can also emulate the slicing [::-1]
using slice(None, None, -one)
. In this case, passing None
to start
and stop
means that you want a slice from the beginning to the end of the target sequence.
Hither's how yous can utilize slice()
to create a reversed copy of an existing list:
>>>
>>> digits = [ 0 , 1 , two , iii , 4 , five , 6 , 7 , 8 , 9 ] >>> digits [ slice ( None , None , - 1 )] [ix, 8, 7, half dozen, 5, 4, iii, 2, 1, 0]
The slice
object extracts all the items from digits
, starting from the right end back to the left terminate, and returns a reversed copy of the target list.
Generating Reversed Lists by Hand
So far, yous've seen a few tools and techniques to either contrary lists in place or create reversed copies of existing lists. Most of the fourth dimension, these tools and techniques are the manner to go when it comes to reversing lists in Python. Yet, if you ever need to opposite lists by mitt, then it'd be beneficial for you to understand the logic behind the process.
In this section, you'll learn how to reverse Python lists using loops, recursion, and comprehensions. The thought is to get a list and create a copy of it in contrary order.
Using a Loop
The first technique you'll use to reverse a listing involves a for
loop and a list concatenation using the plus symbol (+
):
>>>
>>> digits = [ 0 , i , two , iii , four , 5 , 6 , vii , 8 , 9 ] >>> def reversed_list ( a_list ): ... result = [] ... for item in a_list : ... result = [ item ] + consequence ... return issue ... >>> reversed_list ( digits ) [9, 8, 7, 6, five, 4, 3, 2, 1, 0]
Every iteration of the for
loop takes a subsequent particular from a_list
and creates a new list that results from concatenating [item]
and event
, which initially holds an empty list. The newly created listing is reassigned to effect
. This role doesn't modify a_list
.
You lot tin also have advantage of .insert()
to create reversed lists with the assistance of a loop:
>>>
>>> digits = [ 0 , 1 , 2 , 3 , 4 , five , half dozen , 7 , viii , ix ] >>> def reversed_list ( a_list ): ... upshot = [] ... for item in a_list : ... upshot . insert ( 0 , item ) ... render result ... >>> reversed_list ( digits ) [9, viii, 7, 6, five, 4, iii, 2, one, 0]
The call to .insert()
inside the loop inserts subsequent items at the 0
index of upshot
. At the end of the loop, yous get a new listing with the items of a_list
in opposite society.
Using .insert()
similar in the to a higher place example has a meaning drawback. Insert operations at the left stop of Python lists are known to be inefficient regarding execution time. That's considering Python needs to move all the items one footstep back to insert the new item at the outset position.
Using Recursion
You lot can also use recursion to contrary your lists. Recursion is when you define a function that calls itself. This creates a loop that can become infinite if you don't provide a base example that produces a event without calling the part once again.
You lot need the base of operations case to terminate the recursive loop. When it comes to reversing lists, the base case would be reached when the recursive calls get to the end of the input list. You also need to define the recursive instance, which reduces all successive cases toward the base case and, therefore, to the loop's finish.
Here'due south how you tin can ascertain a recursive function to return a reversed copy of a given listing:
>>>
>>> digits = [ 0 , one , two , iii , four , v , six , 7 , eight , ix ] >>> def reversed_list ( a_list ): ... if len ( a_list ) == 0 : # Base case ... return a_list ... else : ... # print(a_list) ... # Recursive case ... return reversed_list ( a_list [ 1 :]) + a_list [: one ] ... >>> reversed_list ( digits ) [9, eight, 7, vi, 5, 4, 3, 2, 1, 0]
Within reversed_list()
, y'all get-go bank check the base case, in which the input list is empty and makes the function return. The else
clause provides the recursive instance, which is a phone call to reversed_list()
itself but with a slice of the original list, a_list[1:]
. This slice contains all the items in a_list
except for the start item, which is then added equally a single-item list (a_list[:1]
) to the result of the recursive telephone call.
The commented call to print()
at the start of the else
clause is just a fob intended to show how subsequent calls reduce the input list toward the base case. Go ahead and uncomment the line to see what happens!
Using a Listing Comprehension
If yous're working with lists in Python, and so you probably want to consider using a listing comprehension. This tool is quite pop in the Python infinite because information technology represents the Pythonic style to procedure lists.
Here's an example of how to use a listing comprehension to create a reversed listing:
>>>
>>> digits = [ 0 , 1 , 2 , 3 , four , five , 6 , 7 , 8 , ix ] >>> last_index = len ( digits ) - i >>> [ digits [ i ] for i in range ( last_index , - one , - i )] [9, viii, 7, half-dozen, 5, 4, 3, 2, 1, 0]
The magic in this list comprehension comes from the call to range()
. In this example, range()
returns indices from len(digits) - i
back to 0
. This makes the comprehension loop iterate over the items in digits
in opposite, creating a new reversed list in the process.
Iterating Through Lists in Reverse
Up to this point, you've learned how to create reversed lists and also how to reverse existing lists in identify, either by using tools specially designed to reach that task or by using your own hand-coded solutions.
In day-to-day programming, yous might detect that iterating through existing lists and sequences in reverse social club, typically known as reverse iteration, is a fairly common requirement. If that's your case, and then you accept several options. Depending on your specific needs, you can utilize:
- The built-in office
reversed()
- The slicing operator,
[::]
- The special method
.__reversed__()
In the post-obit few sections, you'll larn about all these options and how they can help you lot iterate over lists in contrary order.
The Built-in reversed()
Part
Your first approach to iterating over a list in reverse society might be to use reversed()
. This built-in function was specially designed to support opposite iteration. With a list equally an statement, information technology returns an iterator that yields the input list items in reverse guild.
Here's how yous can use reversed()
to iterate through the items in a list in opposite order:
>>>
>>> digits = [ 0 , i , 2 , three , 4 , 5 , 6 , seven , 8 , 9 ] >>> for digit in reversed ( digits ): ... impress ( digit ) ... 9 8 7 6 5 iv iii 2 1 0
The first thing to note in this example is that the for
loop is highly readable. The name of reversed()
clearly expresses its intent, with the subtle item of communicating that the function doesn't produce any side furnishings. In other words, information technology doesn't modify the input list.
The loop is besides efficient in terms of memory usage because reversed()
returns an iterator that yields items on demand without storing them all in memory at the same fourth dimension. Again, a subtle detail to annotation is that if the input listing changes during the iteration, and then the iterator sees the changes.
The Slicing Operator, [::-1]
The second arroyo to reverse iteration is to use the extended slicing syntax you saw before. This syntax does nothing in favor of retentivity efficiency, dazzler, or clarity. Still, information technology provides a quick way to iterate over a reversed copy of an existing list without the risk of being affected by changes in the original list.
Here'south how you can use [::-1]
to iterate through a copy of an existing listing in reverse order:
>>>
>>> digits = [ 0 , 1 , 2 , 3 , iv , v , half dozen , 7 , 8 , nine ] >>> for digit in digits [:: - 1 ]: ... print ( digit ) ... 9 8 7 vi 5 4 3 two 1 0
When yous slice a list similar in this example, you create a reversed copy of the original list. Initially, both lists comprise references to the same group of items. However, if you assign a new value to a given item in the original list, like in digits[0] = "zero"
, then the reference changes to point to the new value. This manner, changes on the input listing don't affect the re-create.
You can have advantage of this kind of slicing to safely alter the original list while you lot iterate over its erstwhile items in opposite society. For case, say you need to iterate over a list of numbers in reverse order and supplant every number with its square value. In this case, y'all can exercise something similar this:
>>>
>>> numbers = [ 0 , 1 , 2 , three , 4 , 5 , 6 , 7 , 8 , 9 ] >>> for i , number in enumerate ( numbers [:: - 1 ]): ... numbers [ i ] = number ** two ... >>> # Square values in reverse gild >>> numbers [81, 64, 49, 36, 25, xvi, 9, 4, 1, 0]
Here, the loop iterates through a reversed copy of numbers
. The call to enumerate()
provides ascending nothing-based indices for each particular in the reversed copy. That allows you to modify numbers
during the iteration. Then the loop modifies the numbers
past replacing each detail with its square value. Every bit a result, numbers
ends up containing square values in reverse order.
The Special Method .__reversed__()
Python lists implement a special method called .__reversed__()
that enables reverse iteration. This method provides the logic backside reversed()
. In other words, a call to reversed()
with a listing as an argument triggers an implicit call to .__reversed__()
on the input listing.
This special method returns an iterator over the items of the current list in reverse order. Even so, .__reversed__()
isn't intended to be used directly. Well-nigh of the time, you'll use information technology to equip your own classes with reverse iteration capabilities.
For example, say you want to iterate over a range of floating-point numbers. Yous can't apply range()
, then you decide to create your own course to approach this specific apply case. You lot end up with a grade similar this:
# float_range.py class FloatRange : def __init__ ( self , start , stop , step = ane.0 ): if offset >= stop : raise ValueError ( "Invalid range" ) self . start = start self . stop = end cocky . step = step def __iter__ ( self ): north = self . start while northward < self . stop : yield north n += self . pace def __reversed__ ( self ): n = cocky . stop - self . pace while due north >= cocky . get-go : yield n n -= cocky . step
This form isn't perfect. Information technology'south just your offset version. However, it allows you to iterate through an interval of floating-bespeak numbers using a fixed increment value, step
. In your class, .__iter__()
provides support for normal iteration and .__reversed__()
supports reverse iteration.
To use FloatRange
, you can practice something similar this:
>>>
>>> from float_range import FloatRange >>> for number in FloatRange ( 0.0 , 5.0 , 0.5 ): ... impress ( number ) ... 0.0 0.5 i.0 i.5 ii.0 two.5 three.0 three.5 4.0 four.5
The class supports normal iteration, which, as mentioned, is provided by .__iter__()
. At present you lot can endeavour to iterate in reverse order using reversed()
:
>>>
>>> from float_range import FloatRange >>> for number in reversed ( FloatRange ( 0.0 , v.0 , 0.5 )): ... impress ( number ) ... 4.v 4.0 3.5 3.0 ii.5 two.0 i.5 1.0 0.5 0.0
In this case, reversed()
relies on your .__reversed__()
implementation to provide the reverse iteration functionality. This way, you lot have a working floating-indicate iterator.
Reversing Python Lists: A Summary
Up to this point, you've learned a lot about reversing lists using different tools and techniques. Hither'south a tabular array that summarizes the more important points y'all've already covered:
Feature | .reverse() | reversed() | [::-1] | Loop | List Comp | Recursion |
---|---|---|---|---|---|---|
Modifies the list in identify | ✔ | ❌ | ❌ | ✔/❌ | ❌ | ❌ |
Creates a re-create of the listing | ❌ | ❌ | ✔ | ✔/❌ | ✔ | ✔ |
Is fast | ✔ | ✔ | ❌ | ❌ | ✔ | ❌ |
Is universal | ❌ | ✔ | ✔ | ✔ | ✔ | ✔ |
A quick look at this summary volition allow you to make up one's mind which tool or technique to use when you're reversing lists in place, creating reversed copies of existing lists, or iterating over your lists in contrary order.
Sorting Python Lists in Reverse Order
Another interesting option when information technology comes to reversing lists in Python is to use .sort()
and sorted()
to sort them in reverse order. To practise that, you can pass True
to their respective reverse
statement.
The goal of .sort()
is to sort the items of a list. The sorting is washed in place, so it doesn't create a new list. If you set the contrary
keyword argument to Truthful
, and then you lot get the list sorted in descending or reverse order:
>>>
>>> digits = [ 0 , 5 , 7 , 3 , 4 , 9 , 1 , half dozen , 3 , 8 ] >>> digits . sort ( opposite = True ) >>> digits [9, 8, 7, half dozen, 5, four, 3, iii, i, 0]
Now your list is fully sorted and also in opposite order. This is quite convenient when you lot're working with some data and yous need to sort it and contrary it at the same time.
On the other paw, if y'all desire to iterate over a sorted list in reverse order, and so y'all can use sorted()
. This built-in function returns a new listing containing all the items of the input iterable in order. If you pass True
to its reverse
keyword argument, then you get a reversed copy of the initial list:
>>>
>>> digits = [ 0 , 5 , vii , three , four , 9 , 1 , 6 , 3 , 8 ] >>> sorted ( digits , opposite = True ) [9, eight, 7, half-dozen, v, iv, three, three, i, 0] >>> for digit in sorted ( digits , reverse = True ): ... print ( digit ) ... 9 8 vii six v four 3 iii 1 0
The reverse
argument to sorted()
allows y'all to sort iterables in descending club instead of in ascending order. So, if y'all demand to create sorted lists in reverse club, then sorted()
is for you.
Decision
Reversing and working with lists in reverse order might be a fairly mutual job in your day-to-day work as a Python coder. In this tutorial, you took advantage of a couple of Python tools and techniques to reverse your lists and manage them in reverse order.
In this tutorial, y'all learned how to:
- Contrary your lists in place using
.reverse()
and other techniques - Use
reversed()
and slicing to create reversed copies of your lists - Use iteration, comprehensions, and recursion to create reversed lists
- Iterate through your lists in reverse order
- Sort lists in contrary order using
.sort()
andsorted()
All of this cognition helps you improve your list-related skills. It provides yous with the required tools to be more skilful when you're working with Python lists.
Source: https://realpython.com/python-reverse-list/
0 Response to "Write a Program. That Reads a Word and Print the Word in Reverse"
Post a Comment